Exploring Python and Tor for IP Address Change: A Guide
Learn how to change your IP address using Python and Tor. This guide simplifies technical concepts for beginners and offers practical tips for online security.
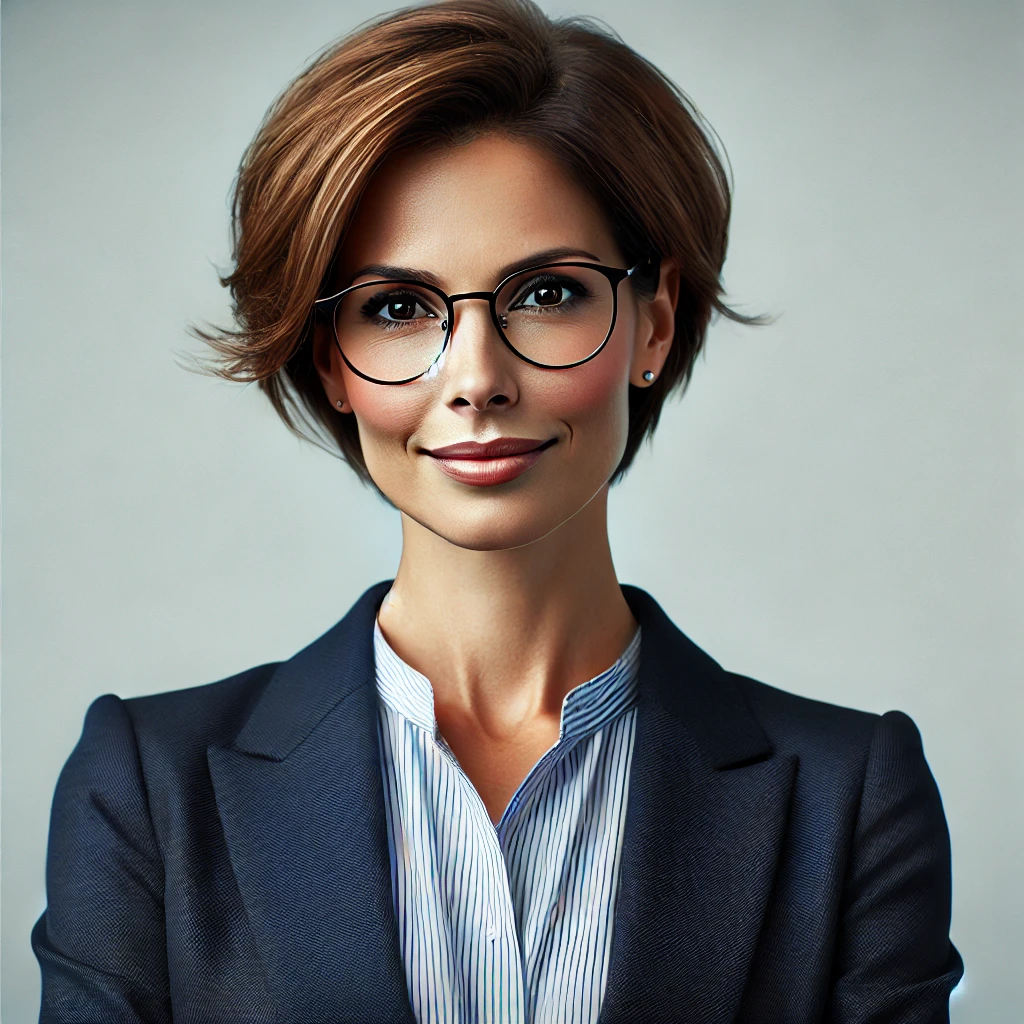
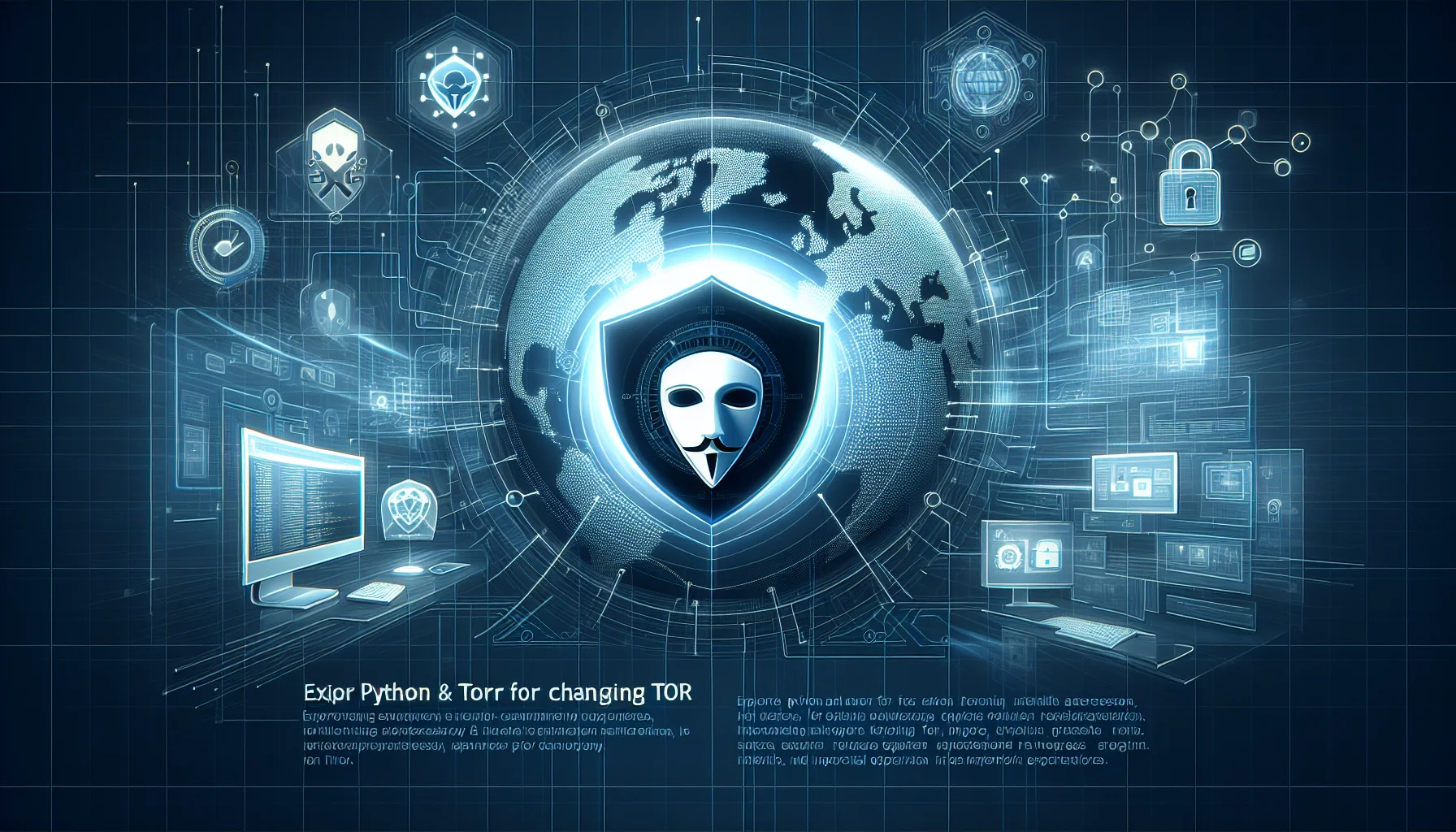
Exploring Python and Tor for IP Address Change: A Guide
Understanding IP Addresses
What is an IP Address?
An IP address is like a home address for your device on the internet. It helps in identifying where data should be sent and received. Imagine your IP address as a postal address for digital information.
Types of IP Addresses
There are two main types of IP addresses: IPv4 and IPv6. IPv4 is like a traditional phone number, consisting of four sets of numbers separated by dots. On the other hand, IPv6 is like a longer phone number that allows for more unique combinations.
Introduction to Python
What is Python?
Python is a popular programming language known for its simplicity and readability. It's like a versatile toolbox that allows you to automate tasks, create applications, and manipulate data. Imagine Python as a Swiss Army knife for coding.
Using Python for Automation
With Python, you can write scripts to automate repetitive tasks, such as renaming multiple files at once or extracting specific information from a large dataset. For example, you can use Python to automatically download the latest weather forecast every morning.
Leveraging Tor for Anonymity
What is Tor?
Tor is a free and open-source software that helps protect your online privacy by routing your internet traffic through a series of relays. It's like wearing a disguise while traveling through secret tunnels to hide your identity.
How Tor Works
When you use Tor, your internet traffic is encrypted and routed through different volunteer-operated servers, making it difficult for anyone to trace your online activity back to you. It's similar to sending a package through multiple couriers, with each courier only knowing the one before and after them.
Practical Implementation
Setting Up the Environment
Before we start implementing our IP changing solution, you'll need to set up your environment:
- Install Python (version 3.6 or higher)
- Install Tor on your system
- Install required Python packages using pip:
pip install requests[socks] stem
Python Code Example
Here's a practical example of how to change your IP address using Python and Tor:
from stem import Signal
from stem.control import Controller
import requests
import time
def get_current_ip():
"""Get the current IP address"""
try:
response = requests.get('https://api.ipify.org?format=json',
proxies={'http': 'socks5h://127.0.0.1:9050',
'https': 'socks5h://127.0.0.1:9050'})
return response.json()['ip']
except Exception as e:
return f"Error: {str(e)}"
def change_ip():
"""Change IP address through Tor"""
try:
with Controller.from_port(port=9051) as controller:
controller.authenticate() # Provide password if set
controller.signal(Signal.NEWNYM)
time.sleep(5) # Wait for new Tor circuit
return True
except Exception as e:
print(f"Error changing IP: {str(e)}")
return False
def main():
print("Initial IP:", get_current_ip())
if change_ip():
print("IP changed successfully!")
print("New IP:", get_current_ip())
else:
print("Failed to change IP")
if __name__ == "__main__":
main()
How to Use the Code
To use this code effectively, follow these steps:
- Ensure Tor service is running on your system
- Enable the control port in your Tor configuration file (torrc):
ControlPort 9051
- Restart the Tor service
- Run the Python script
The script will:
- Show your initial IP address
- Attempt to change it using Tor
- Display your new IP address if successful
Important Security Considerations
When using Python and Tor to change your IP address, keep these security considerations in mind:
- Always download Tor from official sources
- Keep both Tor and Python packages updated
- Avoid hardcoding sensitive information in your scripts
- Be aware that some websites may block Tor exit nodes
- Check for DNS leaks when using proxies
- Use strong authentication for your Tor control port
Enhancing Online Security
Tips for Secure Browsing
- Use strong and unique passwords for each online account to prevent unauthorized access.
- Enable two-factor authentication whenever possible for an extra layer of security.
- Regularly update your software and devices to patch any security vulnerabilities.
- Avoid clicking on suspicious links or downloading attachments from unknown sources.
Importance of Privacy Online
Protecting your online privacy is crucial in today's digital world. By understanding how to change your IP address using Python and Tor, you can take control of your online identity and stay safe while browsing the web.
About the Author
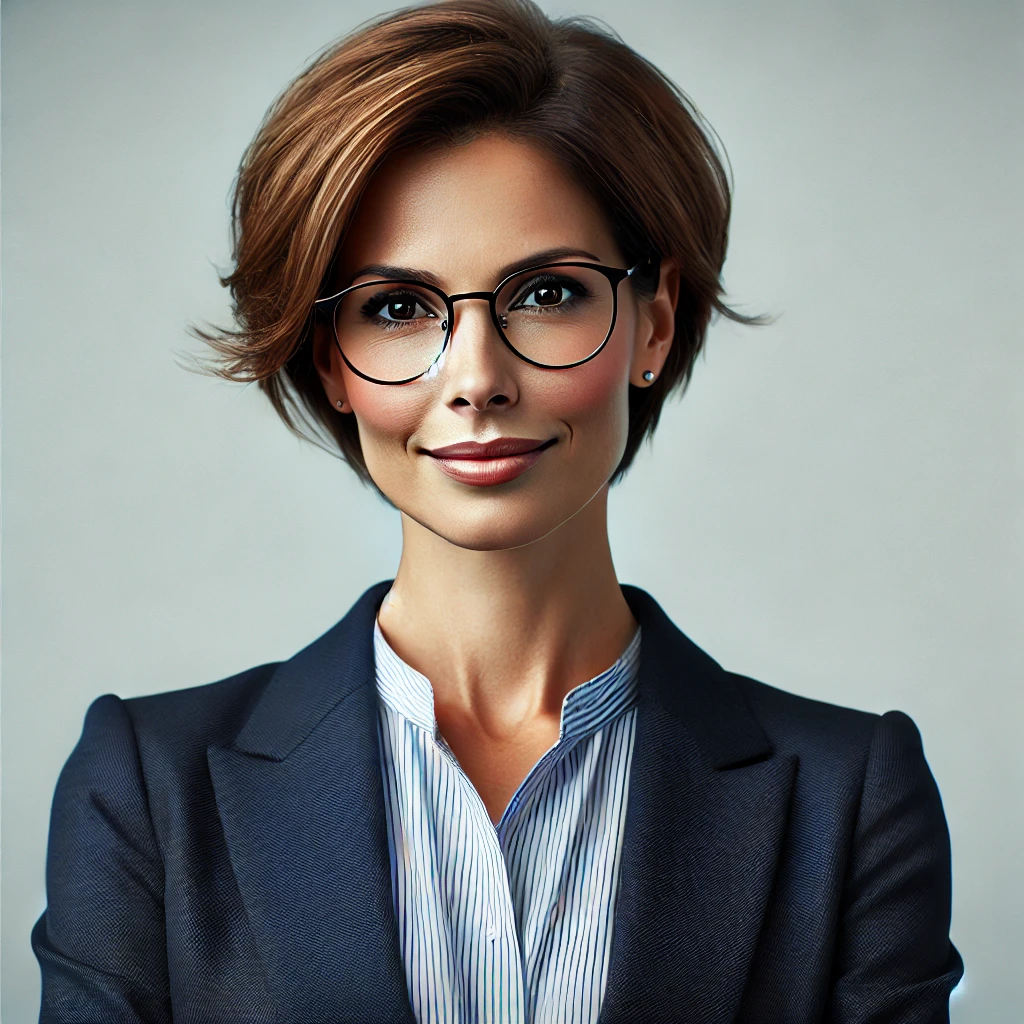
Marilyn J. Dudley
Marilyn is a Senior Network Engineer with over 15 years of experience in network infrastructure design and implementation. She holds CCNA and CCNP certifications and specializes in IP addressing, network security, and IPv6 migration strategies. Throughout her career, she has successfully led numerous large-scale network deployments and IPv6 transition projects for Fortune 500 companies. She is currently a dedicated writer for ipaddress.network, sharing her expertise to help organizations build secure and efficient networks.
Last updated: February 26, 2025